Mastering Switch Statements in Python: A Comprehensive Guide
Written on
Chapter 1: Introduction to Switch Statements
Did you know that Python has introduced the ability to write switch statements? In just five minutes, you can learn how to implement them using code examples.
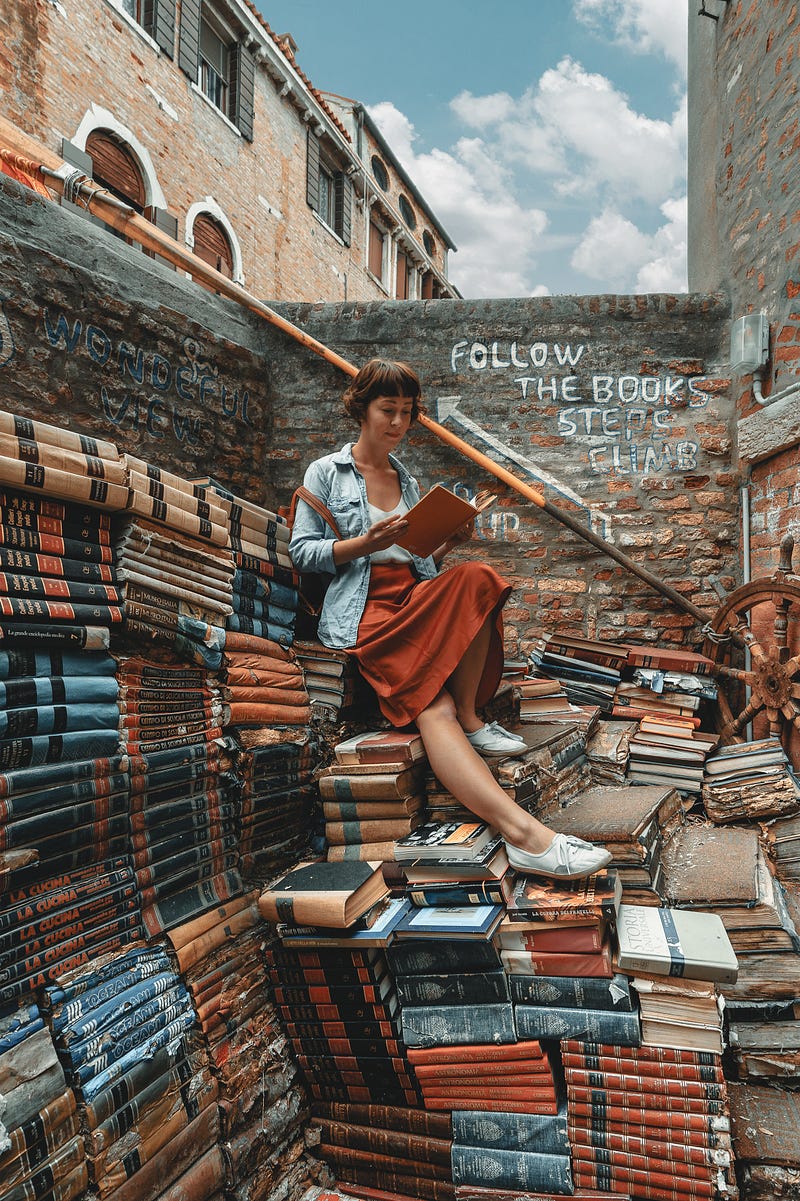
Photo by Clay Banks on Unsplash.
Switch statements are widely favored as an alternative to the if-else structure and are available in many major programming languages like C++, Java, and JavaScript. Until recently, this functionality was absent in Python. With the introduction of version 3.10, Python now supports switch statements through a new keyword: match. Let’s explore how it operates.
Section 1.1: Syntax and Fundamentals
The syntax for the match command resembles traditional switch statements:
match variable:
case pattern:
# execute codecase pattern2:
# execute alternative codecase other:
# fallback for no matches
Key components include a variable to match against, individual patterns defined using the case keyword, and an other clause to handle cases where none of the specified patterns match. Notably, the case other acts similarly to an else statement, and can be simplified to case _, where _ serves as a wildcard for any unmatched pattern.
Let’s review a basic example:
command = "Macbeth"
match command:
case 'Macbeth':
print('Shakespeare!')case 'Emma':
print('Austen')case 'Anna Karenina':
print('Tolstoy')case other:
print("Author not found!")
Output:
Shakespeare!
Now that you grasp the basics of this new feature, let's delve into some advanced use cases.
Section 1.2: Advanced Pattern Matching
Often, you may want to match multiple patterns within a single case. This can be achieved using Python's pipe (|) pattern:
match command:
case 'Macbeth' | 'As You Like It' | 'Othello':
print('Shakespeare!')case 'Emma' | 'Pride and Prejudice':
print('Austen')case 'Anna Karenina' | 'Resurrection':
print('Tolstoy')case other:
print("Author not found!")
Tuples can also be utilized to match combinations of variables and conditions:
weather = ("Saturday", 4)
match weather:
case ("Monday", temp) if temp > 30:
print(f"It's too hot on Monday, {temp=}")case ("Saturday", temp) if temp < 10:
print(f"It's too cold on Saturday, {temp=}")case (weekday, temp) if temp < 18:
print(f"It's slightly chilly on {weekday=}, {temp=}")case (weekday, temp) if temp > 25:
print(f"It's hot on {weekday=}, {temp=}")case _:
raise ValueError("Not a valid weather pattern. Please try again.")
Output:
It's too cold on Saturday, temp = 4
You can also match any item within a list, using lists as case patterns:
match command:
case ['Macbeth', 'As You Like It', 'Othello']:
print("Shakespeare!")case ['Emma', 'Pride and Prejudice']:
print("Austen!")case _:
print("Author not found!")
This behaves similarly to the pipe command discussed previously.
Chapter 2: Conclusion
Congratulations on following along! You’ve learned how the match statement enhances readability and reduces the amount of code needed compared to traditional if-elif-else structures. With this new addition to Python, you can write more concise and efficient code.
Feel free to explore further! If you found this article helpful, consider subscribing to my newsletter for diverse programming insights.
The first video titled "Python gets REAL Switch Case Statement" provides a brief overview of switch statements in Python.
The second video, "MASTER the Match Statement in Python | Python 3.10 Switch Case Complete Guide," offers an in-depth look at the match statement and its applications.