Effective API Error Management in Vue.js Applications
Written on
Chapter 1 Understanding API Errors
In modern web development, Application Programming Interfaces (APIs) play a crucial role in facilitating communication between different services and exchanging data. However, this interaction is not without its challenges. For example, when attempting to retrieve user information through an API, there might be instances where the requested record is unavailable, the user lacks permission to access certain data, or a server error occurs. These potential points of failure must be managed effectively.
If these edge cases are not addressed in frontend applications, users may encounter vague error messages that lead to confusion and a poor user experience. To ensure that users are informed of issues in a clear manner, a well-designed frontend application should consider all possible error scenarios.
This article will delve into practical methods for handling API errors, ensuring that users receive meaningful feedback and guidance to address any problems encountered. Below is an example implementation that effectively manages various edge cases while providing informative notifications for errors.
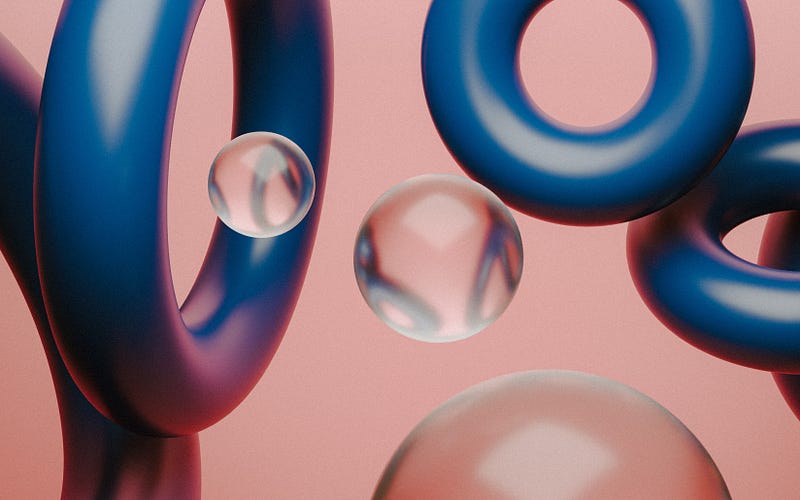
If you're new to setting up toast notifications in a Vue.js application, you might find the following resource helpful:
How to Set Up Toast Notifications in a Vue.js Application
Section 1.1 Best Practices for API Error Handling
When it comes to managing API errors on the frontend, consider the following best practices:
- Implement Catch Blocks: Enclose API requests within try-catch blocks to gracefully handle any exceptions that may arise. This approach prevents the entire application from crashing due to unhandled errors.
- Parse Error Responses: APIs typically return detailed error messages in JSON format. Extract relevant information, such as error messages or codes, to present to users in a clear and friendly manner.
- Use HTTP Status Codes: Pay attention to the HTTP status codes returned by the API. Different codes signal various types of errors (e.g., 404 for not found, 500 for server errors). Ensure your frontend code handles each status code appropriately.
- Show User-Friendly Messages: Display concise and clear error messages that explain what went wrong and offer guidance on how to resolve the issue. Avoid exposing raw technical details to users, as this can be confusing and may pose security risks.
- Log Errors: Keep track of API errors on the client-side to gather useful data for debugging. However, be careful not to log sensitive information in production settings.
- Retry Mechanism: Consider implementing a retry mechanism for transient errors (e.g., network timeouts) to allow the API to recover from temporary issues. Avoid excessive retries to prevent overloading the API.
- Timeouts: Establish reasonable timeouts for API requests to avoid indefinite waiting periods for responses. Provide users with clear messages if requests time out.
- Handle Network Errors: Beyond API-specific errors, also manage network-related issues, such as connection failures or CORS problems. Display relevant messages or encourage users to check their internet connectivity.
- Provide Contact Information: For critical errors, consider offering contact details or support links to help users report issues or seek assistance.
- Localization: If your application is designed for an international audience, make sure error messages are localized to accommodate users in different regions.
- Automated Testing: Implement automated tests to simulate API errors and ensure that your error handling mechanisms function as intended.
- Security Considerations: Be vigilant not to reveal sensitive information in error messages, as this can expose system vulnerabilities.
By adhering to these best practices, you can create a frontend application that efficiently manages errors, preserves a smooth user experience, and offers valuable feedback when issues arise.
Chapter 2 Conclusion
By following the recommended strategies outlined in this article, developers can construct resilient and user-friendly frontend applications that effectively interact with APIs while managing errors in a clear and informative manner.
Your assistance in sharing this information would be greatly appreciated. If you have any questions or suggestions for additional content, feel free to reach out at [email protected] or on Twitter @amhjohnphilip.
More Reads
How to Conditionally Apply Class Attribute Values in Vue.js
How to Configure Environment Variables in Vue.js and Nuxt.js
The first video titled "How to handle errors in Vue.js" provides insights into effectively managing errors in your Vue applications.
The second video, "Vue Handle API Errors | Vue Authentication #8," explores strategies for managing API errors in Vue with a focus on authentication.