Mastering Animations with the React Spring Library: A Comprehensive Guide
Written on
Chapter 1: Introduction to React Spring
The React Spring library simplifies the process of incorporating animations into your React applications. This article will guide you through the basics of using the useSpring hook to create stunning effects.
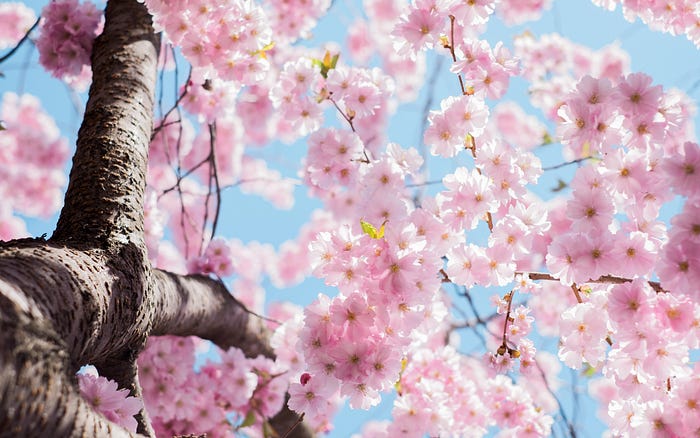
Section 1.1: Getting Started with useSpring
To initiate animations in your app, the useSpring hook is essential. Here’s a basic example illustrating its usage:
import React, { useEffect, useState } from "react";
import { useSpring, animated } from "react-spring";
export default function App() {
const [props, set, stop] = useSpring(() => ({ opacity: 1 }));
const [toggle, setToggle] = useState(false);
useEffect(() => {
set({ opacity: toggle ? 1 : 0 });}, [toggle]);
return (
<>
<animated.div style={props}>I will fade</animated.div>
<button onClick={() => setToggle(!toggle)}>Start</button>
<button onClick={stop}>Stop</button>
</>
);
}
This code snippet demonstrates how to manipulate the opacity of a text element using the set function linked to a toggle state. When the "Start" button is clicked, the text fades in and out accordingly, while the "Stop" button halts the animation.
Section 1.2: Advanced Animation Techniques
You can also use the useSpring hook with an object to define your animations more succinctly:
import React from "react";
import { useSpring, animated } from "react-spring";
export default function App() {
const props = useSpring({
from: { opacity: 0 },
to: { opacity: 1, color: "red" }
});
return (
<>
<animated.div style={props}>I will fade</animated.div></>
);
}
Here, we animate the element's opacity from 0 to 1, along with a color transition to red.
Chapter 2: Async Chains for Dynamic Effects
In this video, we delve into the React Spring library, focusing on how to create basic animations using the useSpring hook.
You can implement asynchronous animations through the to method, allowing for dynamic transitions:
import React from "react";
import { useSpring, animated } from "react-spring";
export default function App() {
const props = useSpring({
to: async (next, cancel) => {
await next({ opacity: 1, color: "#ffaaee" });
await next({ opacity: 0, color: "rgb(14,26,19)" });
},
from: { opacity: 0, color: "red" }
});
return (
<>
<animated.div style={props}>I will fade</animated.div></>
);
}
In this example, the to method is defined as an async function, enabling you to manage the progression of the animation through the next and cancel functions.
This video showcases the implementation of animations using React Spring, elaborating on advanced techniques and practical examples.
Section 2.1: Creating Sequential Animations
You can also create a sequence of animations using an array for the to property:
import React from "react";
import { useSpring, animated } from "react-spring";
export default function App() {
const props = useSpring({
to: [
{ opacity: 1, color: "#ffaaee" },
{ opacity: 0, color: "rgb(14,26,19)" }
],
from: { opacity: 0, color: "red" }
});
return (
<>
<animated.div style={props}>I will fade</animated.div></>
);
}
In this case, the animation will transition through multiple states defined in the array, providing a richer visual experience.
Conclusion
The useSpring hook is a powerful tool that allows developers to create fluid animations for individual elements easily. With this guide, you can begin integrating engaging animations into your React applications, enhancing user experience and interactivity.